How to Work with APIs in JavaScript
- IOTA ACADEMY
- Mar 3
- 4 min read
Updated: Apr 3
Application Programming Interfaces, or APIs, are crucial to contemporary web development. They make it possible for your JavaScript code to control hardware, access data from other platforms, and communicate with external services. Through APIs, you may access social media feeds, integrate payment systems, and retrieve meteorological data. This tutorial will explain what APIs are, their significance, and how to use JavaScript to interact with them.
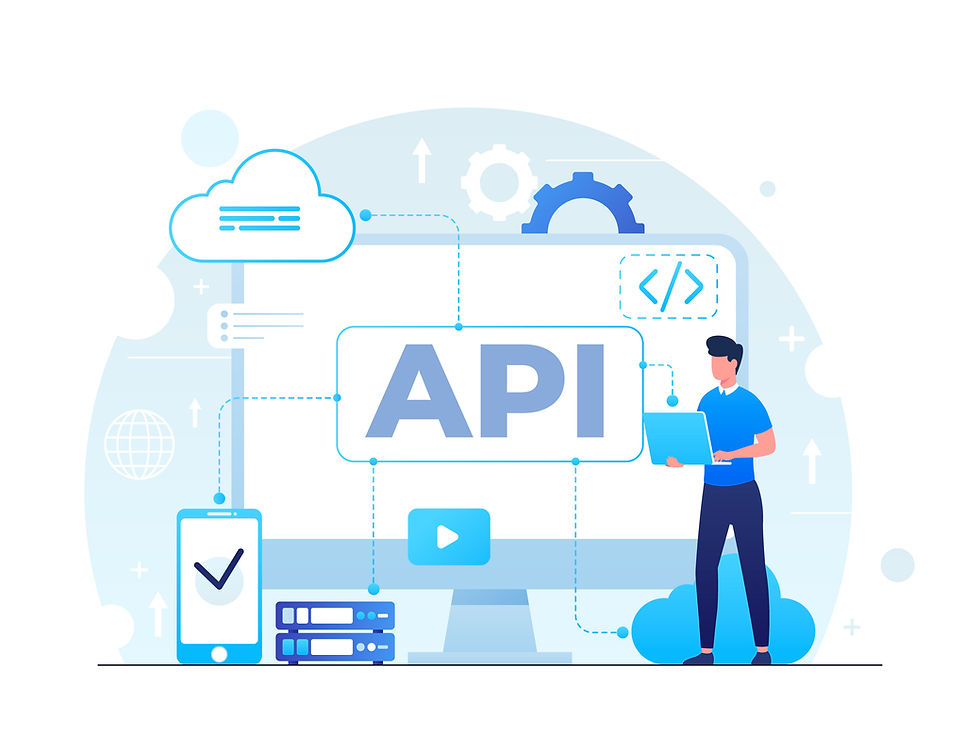
What is an API?
An API is a set of rules that allows one program to communicate with another. Imagine APIs as waiters in a restaurant: you (the user) request a dish, the waiter (API) conveys your order to the kitchen (server), and then delivers the food back to you.
Applications can access data and services from servers thanks to APIs in web development. When you use a weather app, for instance, the app uses an API to retrieve weather data from a server and presents it to you.
Why Use APIs in JavaScript?
JavaScript is the backbone of modern web applications, and APIs enhance its functionality by:
Getting Data from Outside Sources: Retrieve real-time data from Twitter API, GitHub API, and OpenWeather API.
Communicating with Servers: Use AJAX capability to send and receive data without reloading the page.
Automating Tasks: Create programs that communicate programmatically with other services.
Improving User Experience: Offer real-time content changes, such as retrieving fresh comments or posts without requiring a page refresh.
Key Concepts Before Working with APIs
Before diving into code, let’s understand a few essential concepts:
1. HTTP Methods
GET: Retrieve data from an API.
POST: Send data to an API.
PUT: Update existing data.
DELETE: Remove data.
2. Endpoints
An endpoint is a specific URL where an API can be accessed, like https://api.example.com/users.
3. API Keys
Some APIs require an API key for authentication — a unique identifier used to access the API securely.
4. JSON (JavaScript Object Notation)
Most APIs return data in JSON format, which is easy for JavaScript to parse and manipulate.
Tools to Work with APIs in JavaScript
JavaScript offers several ways to work with APIs:
XMLHttpRequest – An older method, not commonly used today.
Fetch API – A modern, built-in JavaScript API for making HTTP requests.
Axios – A popular third-party library with more features and ease of use.
How to Work with APIs Using Fetch API
The Fetch API provides a simple interface for fetching resources, making it the preferred method for most modern JavaScript applications.
Example: Fetching Data from an API
fetch('https://jsonplaceholder.typicode.com/posts') .then(response => response.json()) // Parse JSON data .then(data => console.log(data)) // Log data to console .catch(error => console.error('Error:', error)); |
Explanation:
fetch() sends a request to the provided URL.
.then(response => response.json()) parses the response into JSON format.
.then(data => console.log(data)) processes the JSON data.
.catch() handles any errors that might occur.
Making POST Requests with Fetch
fetch('https://jsonplaceholder.typicode.com/posts', { method: 'POST', headers: { 'Content-Type': 'application/json' }, body: JSON.stringify({ title: 'New Post', body: 'This is the content of the post.', userId: 1 }) }) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error)); |
Explanation:
The method is set to POST.
headers specify the type of content being sent.
body contains the data to be sent, converted to a JSON string.
Using Axios for API Calls
Axios is a promise-based HTTP client for JavaScript, providing additional features over fetch().
Installing Axios
npm install axios |
Example: GET Request with Axios
axios.get('https://jsonplaceholder.typicode.com/posts') .then(response => console.log(response.data)) .catch(error => console.error('Error:', error)); |
Handling API Errors
API calls can fail due to various reasons like network issues or incorrect URLs. Always handle errors using .catch().
Common HTTP Status Codes:
200 OK: Successful request.
400 Bad Request: Incorrect request.
401 Unauthorized: Authentication required.
404 Not Found: Resource not found.
500 Internal Server Error: Server-side issue.
Working with API Authentication
Many APIs require authentication through:
API Keys: Sent as part of the request headers.
OAuth: A more secure method involving tokens.
Example with API Key
fetch('https://api.example.com/data', { headers: { 'Authorization': 'Bearer YOUR_API_KEY' } }) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error)); |
Best Practices When Working with APIs
Read API Documentation: For information on endpoints, methods, and authentication, always consult the API documentation.
Managing Mistakes With Grace: Give error messages that are easy to understand.
Safe API Keys: Your front-end code should never reveal your API keys.
Enhance Performance: Steer clear of pointless cache responses and API queries whenever you can.
Conclusion
Working with JavaScript APIs opens you countless possibilities for your apps, such as integrating third-party services and displaying real-time data. Understanding APIs is essential for any JavaScript developer, regardless of whether they use Axios for more complex requirements or fetch() for simpler requests.
Do you want to gain practical experience with APIs to improve your web development skills? Enroll in the Web development course at IOTA Academy right now! Develop your coding abilities and discover how to create dynamic applications by smoothly integrating APIs.
Enroll right away to get professional help creating interactive web apps!
Comments