Unbalanced datasets, in which one class greatly outnumbers the other, are a common difficulty in machine learning classification challenges. For instance, there are far fewer fake examples in fraud detection than real ones. This mismatch may result in biased models that underperform on the minority class if it is not managed appropriately. This tutorial covers why imbalanced datasets are problematic and effective ways to handle them.
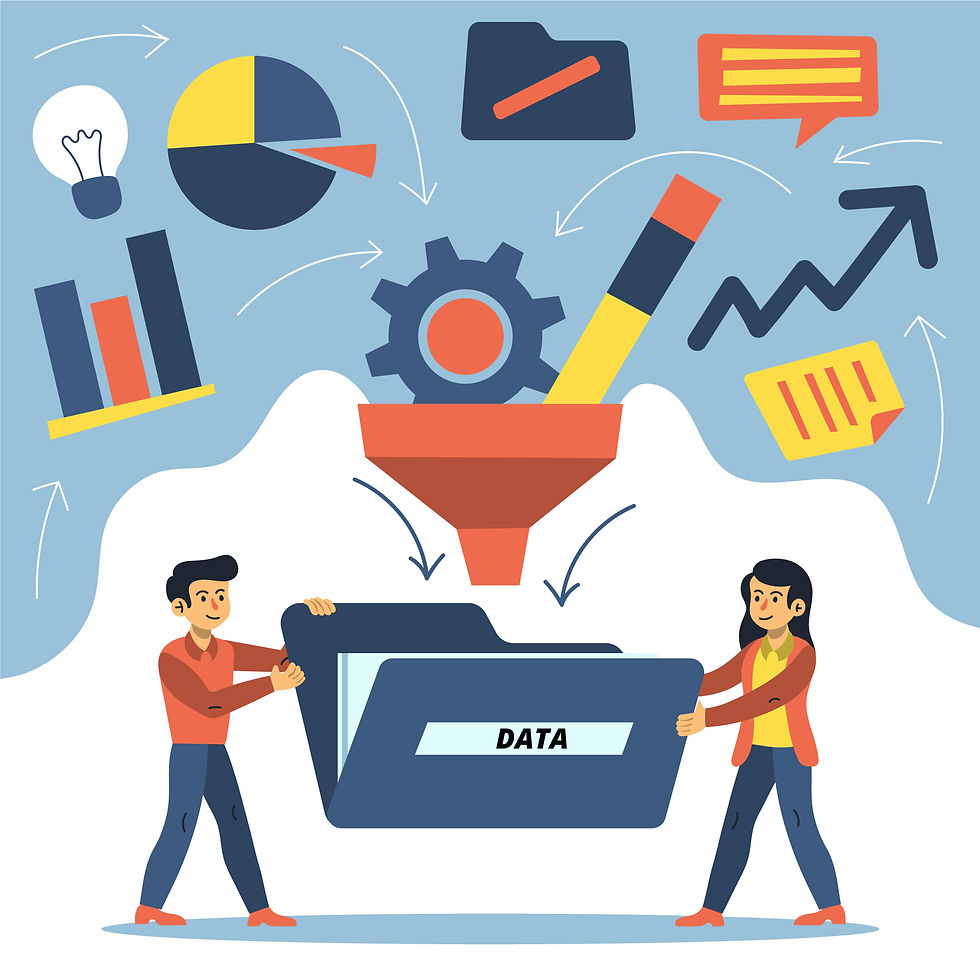
What Are Imbalanced Datasets?
An unequal distribution of classes results in an unbalanced dataset. There are substantially more samples in the majority class than in the minority class.
Example of Imbalanced Datasets:
Fraud Detection: Compared to typical transactions, fraud occurrences are uncommon.
Medical Diagnosis: In medical datasets, rare diseases have fewer positive cases.
Spam Detection: Compared to authentic emails, spam emails are typically less frequent.
In these situations, a model may ignore the minority class, which is frequently more important, and instead concentrate entirely on the majority class.
Why Are Imbalanced Datasets a Problem?
1. Predictions from a biased model: Because it can simply decrease errors by disregarding the minority class, a model trained on an unbalanced dataset typically predicts the majority class.
2. Misleading Accuracy: Even in cases where the model is unable to identify any instances of minority classes, accuracy can nevertheless be high. For instance, if just 5% of transactions are fraudulent, a 95% accurate algorithm might never identify any fraud.
3. Poor Generalization: The model may have trouble correctly predicting new data, particularly for the minority class, which could result in subpar performance in the actual world.
Techniques to Handle Imbalanced Datasets
There are several techniques to manage imbalanced datasets, ranging from modifying the dataset to changing the learning algorithm.
1. Data-Level Techniques
These techniques involve changing the dataset itself, either by adding more samples to the minority class or removing samples from the majority class.

a. Oversampling the Minority Class
To balance the dataset, oversampling entails adding more samples to the minority class. Either making synthetic samples or replicating pre-existing ones can do this. Enough instances of the minority class should be given to the model in order for it to pick up significant trends. Oversampling, however, can occasionally result in overfitting, in which the model learns to recall the duplicated data instead of identifying patterns that can be applied to other situations.
Random Oversampling: Using random oversampling, current samples from the minority class are duplicated at random. Because the same data points are repeated, it raises the possibility of overfitting even if it is straightforward and quick to execute.
from imblearn.over_sampling import RandomOverSampler ros = RandomOverSampler() X_resampled, y_resampled = ros.fit_resample(X, y) |
This method is simple but may lead to overfitting, where the model memorizes duplicate samples instead of learning general patterns.
SMOTE (Synthetic Minority Over-sampling Technique): By interpolating between preexisting samples, the Synthetic Minority Over-sampling Technique, or SMOTE, creates synthetic samples. From the minority class, it chooses two or more cases that are similar, then creates a new sample along the line that connects them. By generating fresh, original data points instead of replicating preexisting ones, this technique lessens overfitting and gives the model more diversity to work with.
from imblearn.over_sampling import SMOTE smote = SMOTE() X_resampled, y_resampled = smote.fit_resample(X, y) |
SMOTE reduces the risk of overfitting by generating new samples instead of duplicating existing ones.
b. Undersampling the Majority Class
In order to balance the dataset, undersampling entails lowering the number of samples in the majority class. When the majority class is big and eliminating a few examples won't have a considerable impact on the model's learning, this method works well. The possible loss of important information when too many samples are destroyed, however, is a significant disadvantage.
Random Undersampling: Until the dataset is balanced, samples from the majority class are randomly eliminated using this technique. Despite being straightforward, it runs the danger of omitting crucial information that could improve the model's generalization.
from imblearn.under_sampling import RandomUnderSampler rus = RandomUnderSampler() X_resampled, y_resampled = rus.fit_resample(X, y) |
This method is simple but may discard important data from the majority class, affecting model performance.
NearMiss: A more advanced undersampling method called NearMiss chooses samples from the majority class that are most similar to the samples from the minority class. NearMiss improves the model's capacity to distinguish between classes by concentrating on the most difficult samples for the model. This guarantees that the data that is preserved is extremely pertinent and instructive.
from imblearn.under_sampling import NearMiss nm = NearMiss() X_resampled, y_resampled = nm.fit_resample(X, y) |
NearMiss ensures that the retained majority samples are highly relevant to the minority class.
2. Algorithm-Level Techniques
These methods modify the learning algorithm to handle class imbalance without changing the dataset.
a. Cost-Sensitive Learning
Different consequences for misclassification errors are introduced via cost-sensitive learning, with the minority class facing harsher penalties. As a result, during training, the model is more likely to focus on the minority class. Cost-sensitive learning can be implemented more easily thanks to algorithms that let you adjust class weights in many machine learning frameworks.
Class Weighting: The algorithm is compelled to lower misclassification mistakes for the minority class by giving it larger weights. This method is very useful since it ensures that the model stays stable and equitable by integrating straight into the learning process without changing the dataset.
from sklearn.ensemble import RandomForestClassifier model = RandomForestClassifier(class_weight='balanced') model.fit(X_train, y_train) |
Class weighting helps the model focus more on the minority class, reducing bias.
b. Ensemble Methods
Several models are combined in ensemble approaches to enhance performance, particularly on minority classes. They lessen the drawbacks of specific models while utilizing the advantages of other models.
Balanced Random Forest: This technique randomly under-samples the majority class for every tree in the forest, balancing the dataset throughout the training stage. A more equitable and accurate model is produced as a result of each tree receiving a balanced selection of data.
from imblearn.ensemble import BalancedRandomForestClassifier model = BalancedRandomForestClassifier() model.fit(X_train, y_train) |
This method ensures that the model is exposed equally to both classes during training.
EasyEnsemble: EasyEnsemble combines ensemble learning and undersampling to produce several balanced subsets of the data. The final choice is determined by combining the predictions of the individual models that were trained on each subset. Because it balances the data without significantly reducing the information from the majority class, this approach is quite effective.
from imblearn.ensemble import EasyEnsembleClassifier model = EasyEnsembleClassifier() model.fit(X_train, y_train) |
EasyEnsemble is effective because it reduces the risk of losing valuable majority class data while balancing the classes.
Evaluating Models on Imbalanced Data
When dealing with imbalanced data, accuracy alone is not a reliable metric. Instead, use the following metrics:
Precision: Measures how many predicted positive cases are actually positive.
Precision is crucial when false positives are costly, such as in fraud detection, where a false positive means flagging a legitimate transaction as fraudulent.
Recall: Measures how many actual positive cases are correctly identified.
High recall is essential when missing positive cases is dangerous, such as in disease diagnosis, where a false negative means failing to detect a disease.
F1-Score: Combines precision and recall into a single metric.
The F1-score is a balanced measure that is useful when both false positives and false negatives are equally important.
AUC-PR (Area Under the Precision-Recall Curve): Measures the trade-off between precision and recall at different thresholds. It provides a comprehensive view of model performance, especially when the dataset is highly imbalanced.
Best Practices for Handling Imbalanced Datasets
Examine Your Information: Recognize the degree of imbalance and how it affects your model.
Try a Variety of Methods: To determine the optimal solution, try both data-level and algorithm-level approaches.
Employ the Proper Metrics: For model evaluation, use precision, recall, F1-score, and AUC-PR.
Cross-Validation: To preserve class distribution across training and testing sets, employ stratified cross-validation.
Conclusion
Building efficient and equitable classification methods requires handling imbalanced datasets. You can enhance your models' performance, particularly on minority classes, by employing strategies like ensemble methods, cost-sensitive learning, oversampling, and undersampling. To make sure your models generalize effectively to new data, always assess them using the proper metrics.
Every data scientist and machine learning practitioner must become proficient in handling unbalanced datasets. Start using these techniques in your projects right now to improve the dependability and performance of your models. Check out our Machine Learning Courses for more in-depth instructions and tutorials to advance your knowledge!
Comments