A Beginner’s Guide to Working with JSON (JavaScript Object Notation)
- IOTA ACADEMY
- 15 hours ago
- 3 min read
JSON (JavaScript Object Notation) has emerged as one of the most popular data communication formats in today's data-driven society. Knowing JSON is crucial whether you're working with databases, online apps, or APIs. A common option in contemporary programming, this lightweight and readable format is made for data storage and transfer.
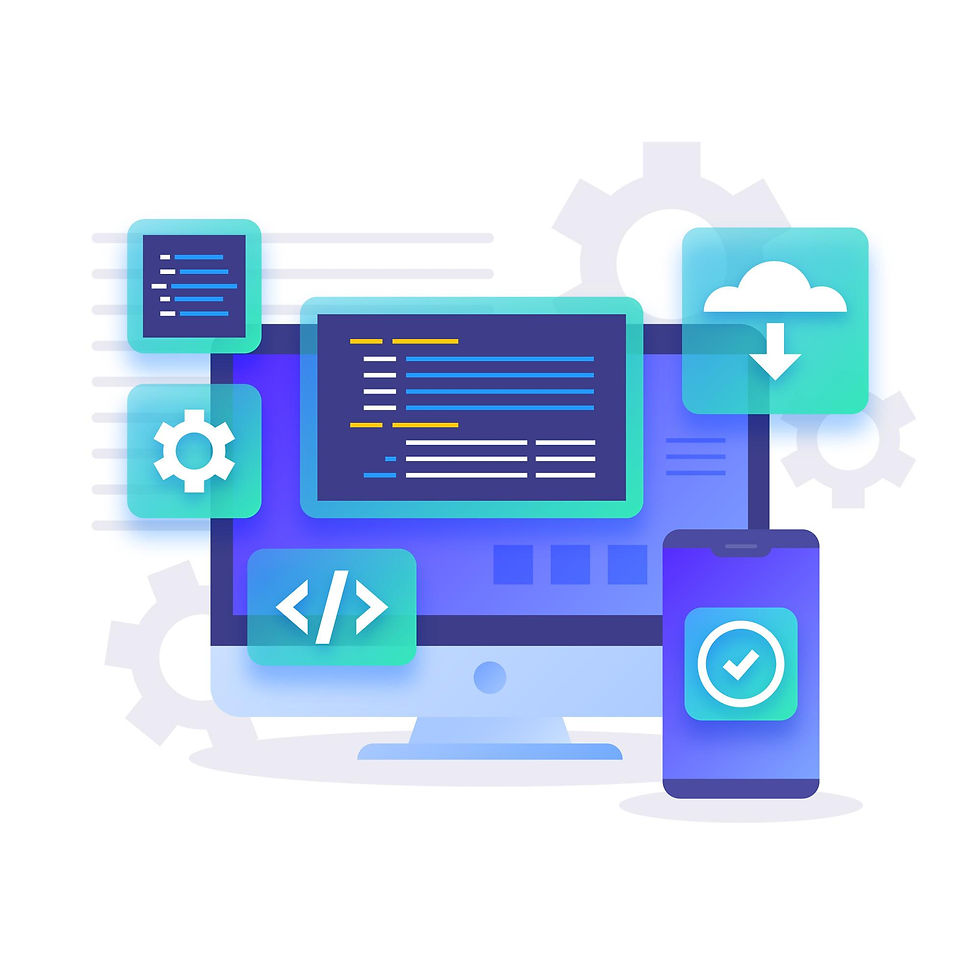
What is JSON?
JSON is a text-based format used to represent structured data. With its key-value pairs, it resembles a dictionary in Python or an object in JavaScript. Because of its ease of usage and compatibility with a wide range of programming languages, JSON is frequently used in web development, data storage, and API connectivity.
Here is an example of a simple JSON object:
{ "name": "John Doe", "age": 30, "city": "New York" } |
In this example, "name", "age", and "city" are keys, and their corresponding values are "John Doe", 30, and "New York". JSON supports different data types, including strings, numbers, booleans, arrays, and nested objects.
Why Use JSON?
For a number of reasons, JSON is favored over other forms like XML. It is easy to read and write because of its straightforward syntax. Because JSON is lightweight, data interchange over the internet can happen more quickly. Additionally, it works with Python, JavaScript, Java, and numerous other programming languages because it is language-independent. Because it offers an organized yet adaptable method of data transmission and reception between apps, JSON is frequently utilized in APIs.
Working with JSON in Python
To work with JSON data, Python comes with an integrated JSON module. JSON.dumps() can be used to convert a Python dictionary to a JSON string, and json.loads() can be used to parse JSON data into a Python object.
Converting Python Dictionary to JSON
import json data = { "name": "Alice", "age": 25, "city": "London" } json_data = json.dumps(data, indent=4) print(json_data) |
This code converts a Python dictionary into a JSON-formatted string. The indent=4 argument makes the output more readable.
Parsing JSON String to Python Dictionary
json_string = '{"name": "Alice", "age": 25, "city": "London"}' parsed_data = json.loads(json_string) print(parsed_data["name"]) # Output: Alice |
This code parses a JSON string and converts it into a Python dictionary, allowing access to individual values using keys.
Reading and Writing JSON Files
JSON is often used to store data in files. You can read and write JSON files in Python using json.load() and json.dump().
Writing JSON to a File
with open("data.json", "w") as file: json.dump(data, file, indent=4) |
Reading JSON from a File
with open("data.json", "r") as file: loaded_data = json.load(file) print(loaded_data) |
These functions allow efficient storage and retrieval of structured data in JSON format.
Handling Nested JSON Data
JSON can have nested structures, meaning values can be objects or arrays. Handling such data requires accessing nested keys correctly.
Example of a nested JSON object:
{ "person": { "name": "Bob", "age": 28, "address": { "street": "123 Main St", "city": "Paris" } } } |
To access nested values in Python:
nested_data = { "person": { "name": "Bob", "age": 28, "address": { "street": "123 Main St", "city": "Paris" } } } print(nested_data["person"]["address"]["city"]) # Output: Paris |
Conclusion
JSON is a crucial data interchange format that is frequently utilized in databases, online apps, and APIs. It is simple to work with in a variety of computer languages because to its lightweight and organized design. You can manage real-world data chores effectively if you know how to read, write, and manipulate JSON data.
Enroll in our course now to improve your data handling abilities and learn JSON!
Comments